DevOps teams are under pressure to deliver faster, more reliable infrastructure—without sacrificing control. This guide shows how DevOps teams automate infrastructure on AWS with Terraform. It combines the flexibility of Infrastructure as Code (IaC) with the scale and control that AWS environments need. You’ll learn how to streamline provisioning, enforce consistency, and reduce manual errors through automation. By the end, you will know how to build cloud infrastructure that is scalable, strong, and cost-effective using Terraform on AWS.
New to Terraform on AWS?
👉Beginner’s Guide to the Terraform AWS Provider
👉3 Benefits of Terraform with AWS
Why DevOps Teams Use Terraform to Automate AWS Infrastructure
For DevOps teams, speed, repeatability, and compliance are non-negotiable. Terraform gives you a way to define AWS infrastructure as code—making it easy to version, peer-review, and automate. Unlike imperative scripts, Terraform uses a declarative approach: you define what the infrastructure should look like, and Terraform handles the how. This simplifies deployments, reduces manual error, and helps teams scale cloud environments predictably.
With broad AWS provider support, teams can codify everything from EC2 instances to IAM policies using HCL (HashiCorp Configuration Language), enabling consistent, auditable infrastructure delivery across environments.
Why DevOps Teams Use the Terraform on AWS Provider
Terraform and AWS are a powerful combination for DevOps teams automating infrastructure at scale. Here’s why it works so well:
- Scalability: Spin up or tear down full environments—including EC2, VPCs, subnets—on demand.
- Consistency: Define infrastructure in .tf files to avoid configuration drift across environments.
- Version Control: Use Git to track changes, enforce reviews, and automate rollbacks.
- Collaboration: Share modules across teams for faster onboarding and less duplicated work.
Setting Up Terraform for AWS Automation: A DevOps Starter
If you’re starting completely from scratch, your first step is to install Terraform on your local machine or wherever you plan to run Terraform. Since the official Terraform Installation Docs provide a clear, step-by-step guide for each operating system, I recommend following those instructions directly.
Once Terraform is set up, you should be able to interact with Terraform CLI. Here are a few prominent commands that you will use with Terraform on a day-to-day basis and their intended actions.
- **terraform version** – Shows you the current version of Terraform that you have installed.
- **terraform -help** – In case of doubt or need to find out about any additional commands, this will show you all available commands and their usage.
- **terraform init** – Initializes the current working directory. It downloads and installs any necessary providers, sets up your backend configuration, and gets everything ready for you to start working.
- **terraform validate** – Checks the syntax of your Terraform configuration files and ensures everything is properly structured. If there’s a missing bracket or an invalid argument, this command will let you know before you go any further.
- **terraform plan** – Generates an execution plan showing what actions Terraform will take based on your configuration (create, update, or delete resources). It’s essentially a “dry run” that helps you visualize proposed changes before actually applying them.
- **terraform apply** – Executes the changes outlined in your plan to create, modify, or destroy resources in your environment. Think of this as the “go” button once you’ve confirmed the plan looks right.
- **terraform destroy** – Tears down and removes the infrastructure managed by your Terraform configuration. It’s a quick way to ensure you don’t leave behind unused resources (and unexpected costs).
- **terraform fmt** – Automatically formats your .tf files according to Terraform’s recommended style conventions. This keeps your code clean and easier for others to read.
- **terraform state** – Provides subcommands for interacting directly with Terraform’s state file. You can use it to inspect resources, remove stale references, or migrate items if needed.
How to Set Up Secure AWS Credentials for Terraform on AWS
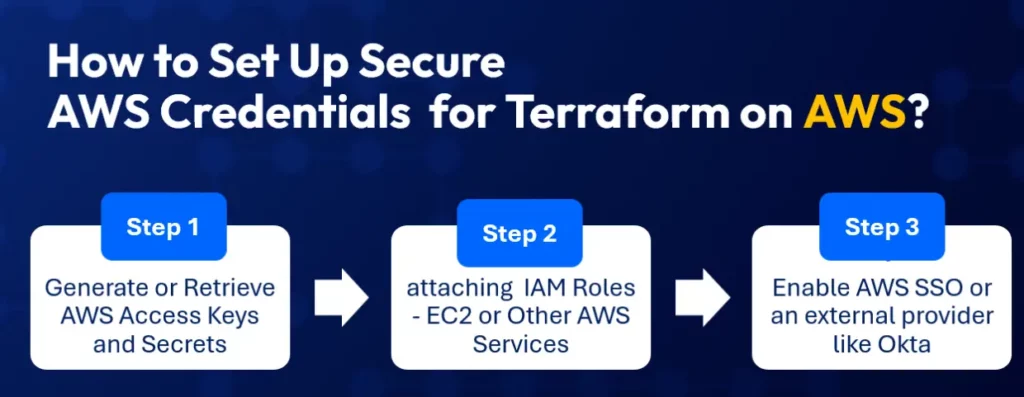
With Terraform installed, you must ensure Terraform can talk to your AWS account. You have several ways to do this; the key is to pick a method that aligns with your security needs and how you plan to run Terraform.
Step 1: AWS Access Keys and Secrets
Generate or Retrieve:
In the AWS Console, head to IAM → Users → Security credentials. Create a new access key if you don’t already have one. You also need to ensure that the particular user has required IAM policies attached to carry out resource management in AWS.
Store Locally (for Testing):
Set these as environment variables:
1 2 3 |
export AWS_ACCESS_KEY_ID="YOUR_ACCESS_KEY" export AWS_SECRET_ACCESS_KEY="YOUR_SECRET_KEY" export AWS_DEFAULT_REGION="us-east-1" |
This is quick and easy if you’re just exploring or running Terraform from a local machine.
Or Use the AWS CLI:
Install the AWS CLI, then run: aws configure
This will create or update your ~/.aws/credentials and ~/.aws/config files. Terraform automatically picks up these files.
Tip: Never commit your AWS keys or secrets to source control. For serious projects, rely on more secure methods (like IAM roles or Vault) to safeguard credentials.
Step 2: IAM Roles (for EC2 or Other AWS Services)
If you’re running Terraform from an AWS EC2 instance, attaching an IAM role to that instance is the most straightforward method.
- Create an IAM Role: Assign the necessary policies (e.g., permissions to create S3 buckets, manage EC2, etc.).
- Attach the Role to Your EC2: Under Actions → Security → Modify IAM role for your running instance.
- Automatic Pickup: Terraform will detect and use these credentials without you having to store any secrets on your machine.
This is also a good approach if you use services like AWS CodeBuild, AWS CodePipeline, or Fargate to run your Terraform commands in a CI/CD pipeline.
Step 3: AWS SSO or Federated Identity Providers
In larger organizations, you might rely on AWS Single Sign-On (SSO) or an external provider like. That way, your team members log in once and assume roles that grant only the privileges they need.
- Enable AWS SSO: Configure SSO in the AWS console, linking it to your identity provider.
- Use the AWS CLI: With AWS CLI v2, you can sign in using aws sso login –profile <your-sso-profile>.
- Run Terraform: Terraform leverages your authenticated SSO session without needing static credentials.
This approach helps enforce short-lived sessions and scope each user’s access more precisely.
How to Configure the Terraform AWS Provider
Provider configuration is how you tell Terraform that you want to manage AWS resources using Terraform. You can add in your AWS credentials as parameters within the provider configuration block. If its not defined, it will automatically pick variables based on the methods that you have configured above.
Create a main.tf file in your Terraform project folder to define the AWS provider.
1 2 3 |
provider "aws" { region = "us-east-1" } |
Be sure to select the AWS region closest to your users or best suited for your workload. We will talk about these .tf files more in the following sections.
Infrastructure as Code (IaC) with Terraform AWS Provider
In Terraform, you define your infrastructure resources using declarative .tf files. Here’s a basic example of creating an EC2 instance: This example obtains the latest AMI (Amazon Machine Image) for the EC2 instance using a Terraform Data block.
Tip: Data configuration block in Terraform is used to get information from existing resources or external sources.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
# Dynamically fetch the latest Ubuntu 22.04 (Jammy) AMI data "aws_ami" "ubuntu_latest" { most_recent = true # Canonical's AWS account ID for official Ubuntu images is 099720109477 owners = ["099720109477"] filter { name = "name" values = ["ubuntu/images/hvm-ssd/ubuntu-jammy-22.04-amd64-server-*"] } } resource "aws_instance" "demo_server_single" { ami = data.aws_ami.ubuntu_latest.id instance_type = "t2.micro" # Basic example user_data to confirm the instance boots user_data = <<-EOF #!/bin/bash echo "Hello from Terraform + Ubuntu!" > /home/ubuntu/hello.txt EOF } |
- Resource Blocks: Used to describe an infrastructure component (like an EC2 instance) in detail. You can provide different arguments like AMI ID, instance type, tags, etc, to describe the resource in more detail. Read more on aws_instance resource.
- Declarative Config: Specify the desired state (e.g., “I want an EC2 instance with these properties”). Terraform handles the provisioning logic for you.
Once you are ready to apply these changes to infrastructure, you can use terraform planto foresee what changes will be done to the infrastructure, and thenterraform applyto apply those changes.
Terraform on AWS State Management: Remote Backends
Terraform uses a state file to keep track of resources you’ve created. This file is crucial because it maps real-world infrastructure to your configuration.
- Local State: By default, Terraform stores state locally (in terraform.tfstate). This might work for personal projects, but it’s not recommended for team scenarios.
- Remote State: Using a remote backend like AWS S3 (often with a DynamoDB table for state locking) is a best practice for team-based projects. This prevents conflicts if multiple people run Terraform at the same time.
Note that remote backend types are not limited to AWS S3 or local. There are several other options that you can use. Read more about those here.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
terraform { # You can pin a Terraform version range for consistency required_version = ">= 1.0" # Storing state in an S3 bucket with DynamoDB table locking backend "s3" { bucket = "demo-terraform-state-bucket" key = "demo-project/terraform.tfstate" region = "us-east-1" dynamodb_table = "demo-terraform-lock-table" # You must create this table separately encrypt = true } } |
Ensure you enable versioning on your S3 bucket and set up a DynamoDB table for state locking to prevent concurrent writes.
Terraform Modules on AWS How to simplify Infrastructure
Terraform modules let you encapsulate and reuse infrastructure configurations. Instead of duplicating code across multiple projects, you can create a module that includes, for example, a VPC with subnets and attach it wherever needed.
You can also store these modules in a version control system and refer to those directly.
- Creating a Module: Put related .tf files in a directory and define input/output variables.
- Using a Module: Reference it with a module block.
The following example uses an official AWS VPC Terraform Module from the Terraform registry to create a new VPC in your AWS account’s specified region instead of using your own. But it’s the same concept.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
# Using official Terraform Module, You can create your own too module "vpc" { source = "terraform-aws-modules/vpc/aws" version = "~> 4.0" # Use a module version compatible with your Terraform setup name = "MyDemoVPC" cidr = "10.0.0.0/16" # Availability Zones azs = ["us-east-1a", "us-east-1b"] # Subnets public_subnets = ["10.0.1.0/24", "10.0.2.0/24"] private_subnets = ["10.0.3.0/24", "10.0.4.0/24"] # NAT Gateways enable_nat_gateway = true single_nat_gateway = true # Tags for the VPC and all child resources tags = { Terraform = "true" Environment = "dev" } } |
This approach keeps your main configuration files clean and organized, making large-scale infrastructure much easier to maintain.
Terraform on AWS: Scaling Infrastructure the Right Way
One of the major advantages of cloud computing is the ability to dynamically scale resources. AWS is known for its highly scalable and reliable backbone cloud infrastructure. Combining Terraform with AWS allows you to even define the ability to scale resources dynamically as Infrastructure as Code (IaC). A few examples are,
- Auto Scaling Groups (ASGs): Use Terraform to manage ASGs for EC2 instances. You can define scaling policies triggered by CloudWatch alarms to match changes in usage demands.
- Load Balancers: Attach an Application Load Balancer (ALB) or Network Load Balancer to route incoming traffic across healthy instances. Terraform makes it straightforward to update load balancer rules or integrate SSL certificates.
- Serverless: Terraform supports AWS Lambda, so you can manage functions and integrate them with triggers like API Gateway or S3 events.
Here’s an example snippet for creating an Auto Scaling Group using Terraform. Note that the values are referred to from previously created resources like VPC and the AMI Image ID.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
esource "aws_security_group" "demo_sg" { name = "demo-sg" vpc_id = module.vpc.vpc_id ingress { from_port = 22 to_port = 22 protocol = "tcp" cidr_blocks = ["0.0.0.0/0"] } egress { from_port = 0 to_port = 0 protocol = "-1" cidr_blocks = ["0.0.0.0/0"] } } resource "aws_launch_template" "demo_lt" { name_prefix = "demo-lt-" image_id = data.aws_ami.ubuntu_latest.id instance_type = "t2.micro" vpc_security_group_ids = [aws_security_group.demo_sg.id] } resource "aws_autoscaling_group" "demo_asg" { name = "demo-asg" max_size = 3 min_size = 1 desired_capacity = 2 vpc_zone_identifier = module.vpc.public_subnets launch_template { id = aws_launch_template.demo_lt.id version = "$Latest" } } |
Terraform Deployments with CI/CD Pipelines
To fully automate and streamline your infrastructure deployments, consider integrating Terraform with a CI/CD pipeline:
- GitHub Actions: Trigger Terraform runs every time you merge to a specific branch.
- AWS CodePipeline: A native AWS solution for continuous integration and delivery that can incorporate Terraform as a build or deploy action.
- ControlMonkey: Helps manage Terraform deployments by providing governance, drift detection, and automation for infrastructure changes.
Regardless of the tool, the goal is to ensure that any changes to your .tf files automatically go through version control, tests, and approvals before they’re applied to production environments.
Terraform Security Best Practices for AWS Deployments
Security is non-negotiable for DevOps teams. A few pointers to keep in mind when using Terraform to manage your AWS cloud infrastructure:
- Manage Secrets and Sensitive Data: Avoid embedding sensitive information (like passwords or private keys) in your .tf files. Use AWS Systems Manager Parameter Store or Vault to securely store these values.
- Least Privilege IAM Policies: Ensure Terraform runs with the minimum IAM permissions. Overly permissive policies increase your attack surface.
- Encrypted State and Traffic: Always enable S3 bucket encryption for state files. If you’re moving data across networks, ensure it’s encrypted in transit (TLS/SSL).
- Proper Network Isolation: If your workloads demand high security, look into private subnets, NAT gateways, and strict security group rules defined via Terraform.
Monitoring and Cost Optimization
Visibility into resource utilization and cost is essential for AWS environments:
- AWS Budgets and Cost Explorer: Set up budgets or alerts for monthly spending thresholds. Terraform can automate the creation of budgets and alerts.
- CloudWatch Metrics and Alarms: Keep track of CPU, memory, and other key metrics. You can also define CloudWatch alarms in Terraform to trigger notifications or scaling actions.
Example of setting CloudWatch alarms via Terraform:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
esource "aws_cloudwatch_metric_alarm" "high_cpu" { alarm_name = "high_cpu_usage" comparison_operator = "GreaterThanOrEqualToThreshold" evaluation_periods = 2 metric_name = "CPUUtilization" namespace = "AWS/EC2" period = 300 statistic = "Average" threshold = 80 alarm_description = "Alarm when CPU exceeds 80%" actions_enabled = true alarm_actions = [aws_sns_topic.alerts.arn] dimensions = { InstanceId = aws_instance.demo_server_single.id } } |
Tagging Strategy with DevOps Terraform AWS
Apply standardized tags (e.g., Environment, Owner) to resources. This helps with cost allocation and environment organization, especially when multiple teams share the same AWS account. Terraform allows you to ensure this is consistent across all your cloud resources and even add additional validation configurations as guard rails to ensure required tags are added and to abort if any tags are missing. This can be done using Terraform custom conditions.
Example of setting custom conditions to ensure whether a required set of tags is specified before creating a resource.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
variable "common_tags" { description = "A map of tags that must be applied to all resources." type = map(string) validation { condition = ( contains(keys(var.common_tags), "Environment") && contains(keys(var.common_tags), "Owner") ) error_message = "You must provide both 'Environment' and 'Owner' keys in the common_tags variable." } } locals { common_tags = { Environment = "dev" Owner = "team-xyz" } } resource "aws_s3_bucket" "demo-bucket" { bucket = "demo-bucket" # We apply the validated tags here tags = var.common_tags } |
Final Thoughts on DevOps Terraform AWS
Terraform and AWS are powerful for DevOps teams aiming to upscale their infrastructure management strategy with Infrastructure as Code (IaC).
Here’s a quick recap of the steps to get started:
- Install and Configure: Get Terraform, configure AWS credentials, and define the AWS provider.
- Write Declarative Configurations: Use .tf files to describe your infrastructure.
- Manage State Properly: Store your state in a remote backend like S3 with DynamoDB locking.
- Adopt Modules: Keep your code DRY (Don’t Repeat Yourself) by leveraging modules.
- Automate with CI/CD: Integrate Terraform runs into your deployment pipelines for greater consistency and speed.
- Secure and Monitor: Adhere to least-privilege IAM policies, enable encryption, and stay on top of costs.
- With these practices in place, you’ll be well on your way to delivering robust and efficient infrastructure at scale using both the Terraform AWS Provider and Terraform on AWS together.
- Don’t forget to destroy any test resources with terraform destroy once you’re done with testing to avoid unexpected billing. Good luck, and happy building!
Take your infrastructure automation to the next level. With ControlMonkey you can streamline Terraform deployments on AWS using AI-powered code generation, automatic drift detection, smart remediation, and robust compliance enforcement across multi-cloud environments. Eliminate manual errors, accelerate provisioning, and ensure every deployment is production-grade.

Frequently Asked Questions (FAQ)
Storing state in S3 (or any remote backend) is optional but highly recommended for team-based or production scenarios. It prevents conflicts if multiple people run Terraform at the same time and provides versioning for your state file.
Yes. Terraform is highly modular. You can choose to manage just your EC2 instances and RDS databases, for example, and still provision other services manually in the AWS Console if you prefer.
Avoid storing secrets directly in .tf files. Use AWS Systems Manager Parameter Store, AWS Secrets Manager, or HashiCorp Vault to keep sensitive data secure. This helps prevent accidental exposure in source code repositories.
If you’re using local AWS access keys, you can generate new keys and update your environment variables or AWS CLI config. For IAM roles or AWS SSO, Terraform automatically picks up the new session tokens after you re-authenticate.
Absolutely. Many teams set up pipelines to run terraform plan and terraform apply whenever they push changes to a repository. This promotes consistent deployments and thorough infrastructure reviews.
You can, but it’s generally best to stick with one Infrastructure as Code solution to keep things consistent. If you already have CloudFormation stacks, you might manage them separately or consider migrating them to Terraform over time.
Running terraform destroy removes all resources defined in your Terraform configuration. This is handy for test environments so you don’t incur costs for idle resources.
Use a combination of modules and environment-specific inputs (via .tfvars files or workspaces). Modules let you standardize and reuse core infrastructure patterns, while each environment’s parameters (like CIDR blocks or instance counts) can be passed in separately. This avoids code duplication and ensures consistent configurations across all environments.
Storing your .tf files in a Git-based repository is ideal. You can review changes via pull requests, track history, and enforce quality checks with CI/CD. Tagging releases or using branches for different environments is also a common practice.
Yes. You can configure multiple providers or use different profiles. Each provider block can point to a distinct region or AWS account, letting you manage complex, distributed infrastructure from a single Terraform configuration.