Terraform Errors are more common than most teams realize. While terraform has become the IaC tool of choice for many organizations. The reality is that Terraform makes it deceptively easy to get started but considerably more challenging to get right. Many teams discover this only after they’ve accumulated significant technical debt. Simple deployments can quickly become maintenance nightmares when you overlook best practices.
This article covers the most frequent issues teams encounter, with practical examples from AWS and Azure. Whether you use a single cloud or multiple clouds, this guidance will help you avoid mistakes. It will also strengthen your infrastructure and improve your Terraform workflow.
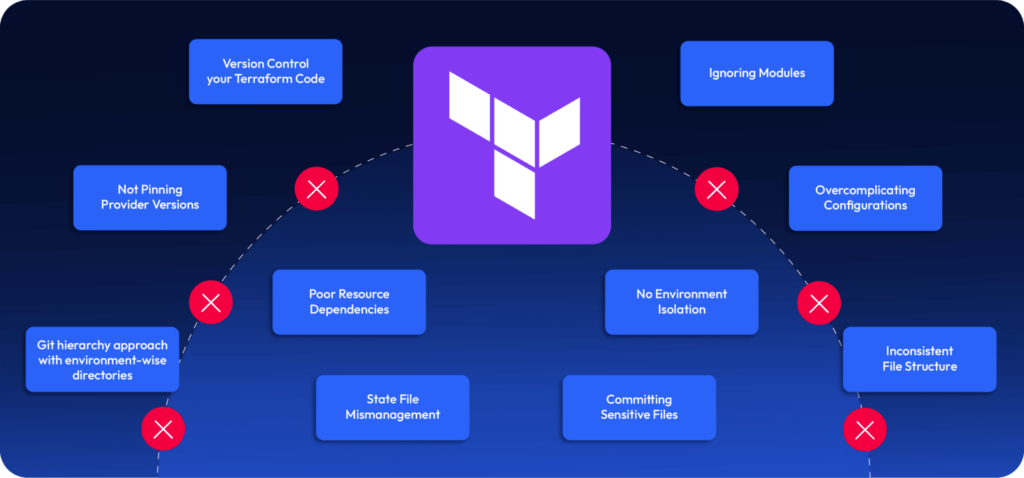
1. First Terraform Error: Version Control your Terraform Code
The benefit of using Terraform to manage infrastructure is that you keep your entire infrastructure configuration declaratively coded in a set of *.tf files. That’s your single source of truth. Whenever you or your team needs to spin up a new environment or troubleshoot an existing one, you look in one place.
With Git, you get a history of how your infrastructure changed and who was responsible for those changes & roll back if necessary. Even a small code change such as a Terraform AWS Provider version upgrade can have a large blast radius. Without version control, it’s impossible to track such changes. A good version control strategy allows teams to collaborate without overriding each other’s work, peer-review their work, and roll back changes if necessary.
Adopt Trunk-Based Development for Better Terraform Collaboration
In a typical application source code repository, most developers adopt the “GitFlow” branching strategy, which has two or more long-lived branches (E.g., main, staging, develop, etc.). Each branch contains a version of the application that will be merged into the upper branch when tested thoroughly and ready for release.
However, unlike application code, infrastructure can have only one version deployed. Keeping multiple long-lived branches in a Terraform repository is not common practice. When handling multiple environments, we manage those environments based on a directory structure or with Terraform workspaces, which we will get to later in this article.
Trunk-based development is a branching strategy where developers merge their changes directly to a main branch, referred to as the “trunk”. The developers ensure that changes are small, focused, and easier to review and validate.
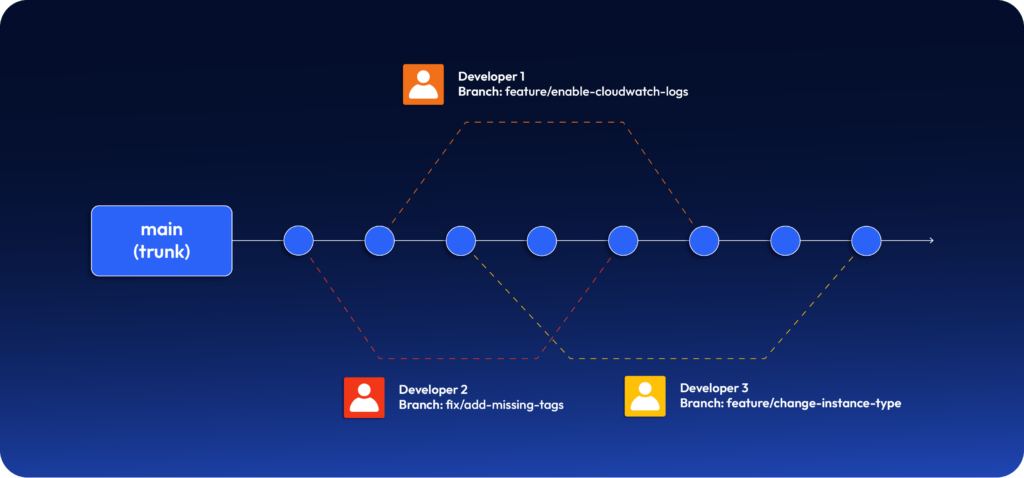
Does that mean we can only have one branch?
In theory, yes. Actually? No.
Based on the source code hosting platform you use (GitHub, GitLab, etc), you can set different merge/commit rules for each branch. For the main branch (trunk), disable direct commits and require peer approval before merging PRs. However, you can have Terraform scripts for test environments in a different branch (say, “test”), which allows direct commits by any developer. But you NEVER merge your test branch to the trunk!
Note: The test branch should not deviate too much from the infrastructure in the main branch. To ensure this, you can routinely compare the production and test setup and recreate the test environment if necessary.
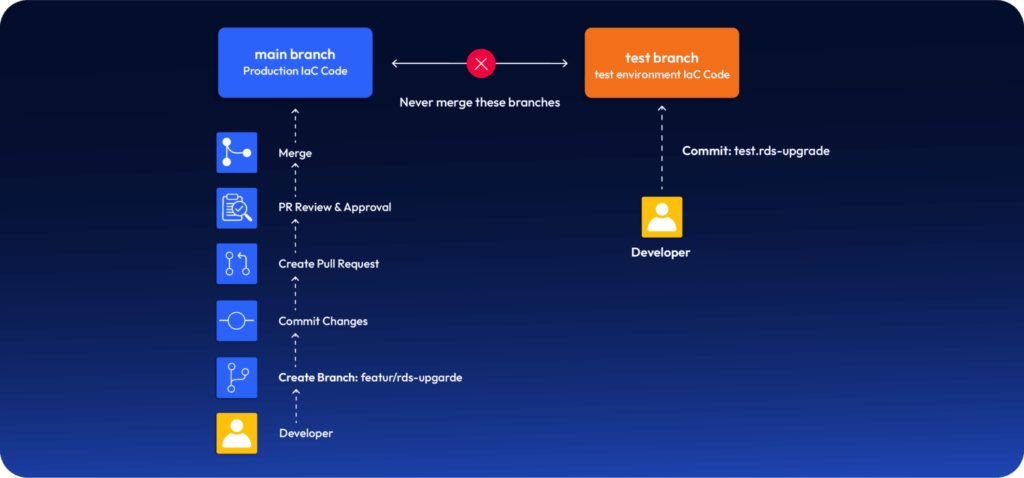
2. Terraform Error: Ignoring Modules in Your Infrastructure
Without modules, lengthy and duplicated code appears across multiple environments as developers copy and paste configurations rather than reusing established patterns. It can cause inconsistencies across environments, and making a simple change would require updates in multiple places. Modules help keep provider versioning such as Terraform AWS provider or Terraform Azure provider consistent across your configuration.
Start your module strategy by identifying repeated patterns in your infrastructure. Common examples include standardized networking components, storage solutions, or security configurations. Design modules with clear, focused purposes—each module should do one thing well rather than trying to address multiple concerns.
Keep these things in mind;
- Keep your module code separate from your primary Terraform infrastructure scripts. Have them in separate git repositories so you can easily version control them and use them in multiple projects.
- Use well-documented and maintained third-party modules in your code. Build wrapper modules around them to incorporate your organizational standards.
3. Not Pinning Provider Versions: A Common Terraform Pitfall
When you don’t specify exact provider versions, Terraform automatically pulls the latest version during initialization, which can lead to unexpected behaviour or broken deployments when providers release breaking changes.
Here is the right way:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
terraform { required_providers { aws = { source = "hashicorp/aws" version = "= 3.74.0" } } } provider "aws" { region = "us-west-2" } terraform { required_providers { azurerm = { source = "hashicorp/azurerm" version = "= 3.76.0" } } } provider "azurerm" { features {} } |
You have several options for version constraints:
- Exact version: version = “= 3.74.0”
- Greater than: version = “> 3.74.0”
- Range constraint: version = “>= 3.74.0, < 4.0.0″
A best practice is to use a pessimistic constraint operator (~>), which allows only the rightmost version component to increment.
4. Terraform Mistake: Poor Resource Dependencies
Terraform builds its dependency graph based on explicit references between resources. But some dependencies exist at runtime that aren’t visible in configuration. Failing to declare these “hidden” dependencies can lead to subtle, hard-to-debug issues where resources are technically created but don’t function properly together.
The example below shows why Terraform can miss important runtime dependencies and how depends_on can be used to fix it:
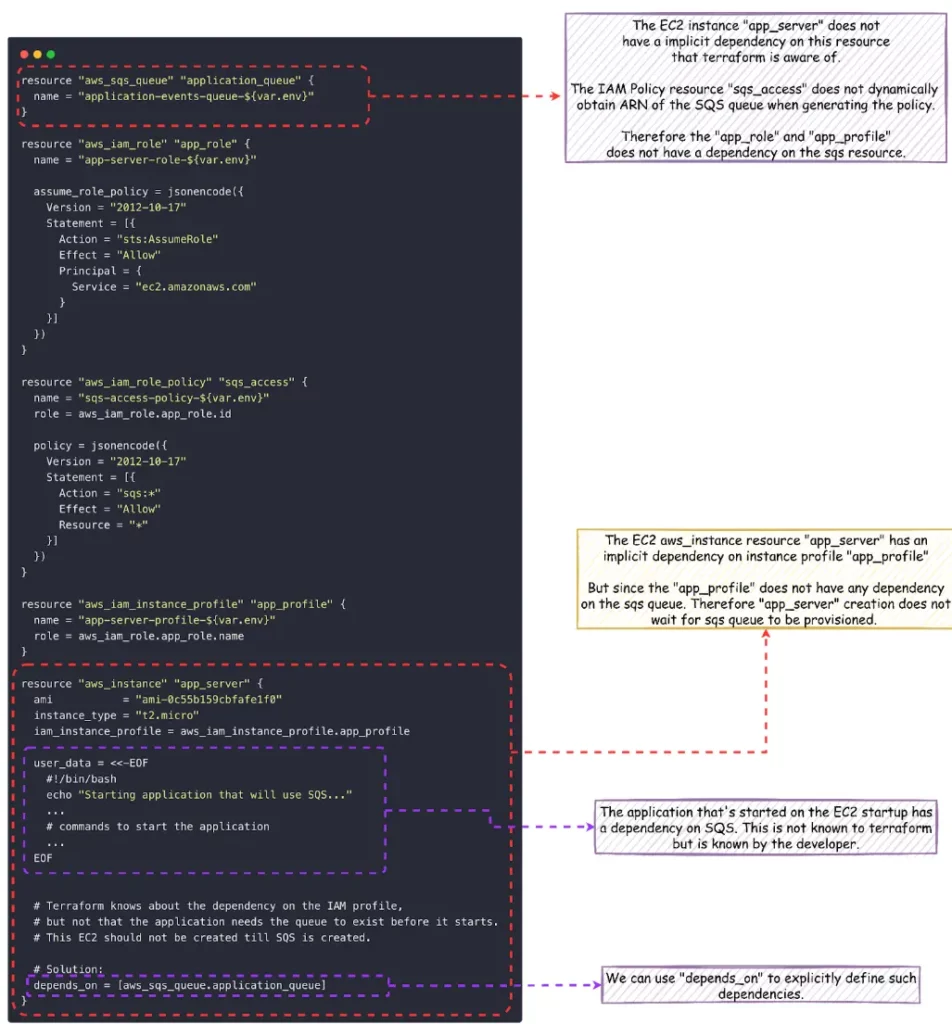
5. No Environment Isolation
What we are talking about here is state file isolation and configuration isolation.
Using the same state file for all environments creates significant risks, including accidental changes during testing and difficulty tracking environment-specific configurations. Similarly using a monolithic configuration leads to complex conditional logic to isolate environments.
👎 Don’t maintain your code as follows, where you use a variable to conditionally deploy resources and use a single state file for all environments;
Instead, you can follow one of the following options;
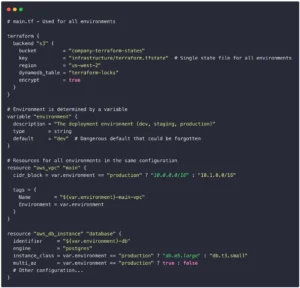
5.1 – Git hierarchy approach with environment-wise directories
The most common approach is to maintain completely separate Terraform configurations for each environment, organized in a clear git directory structure:
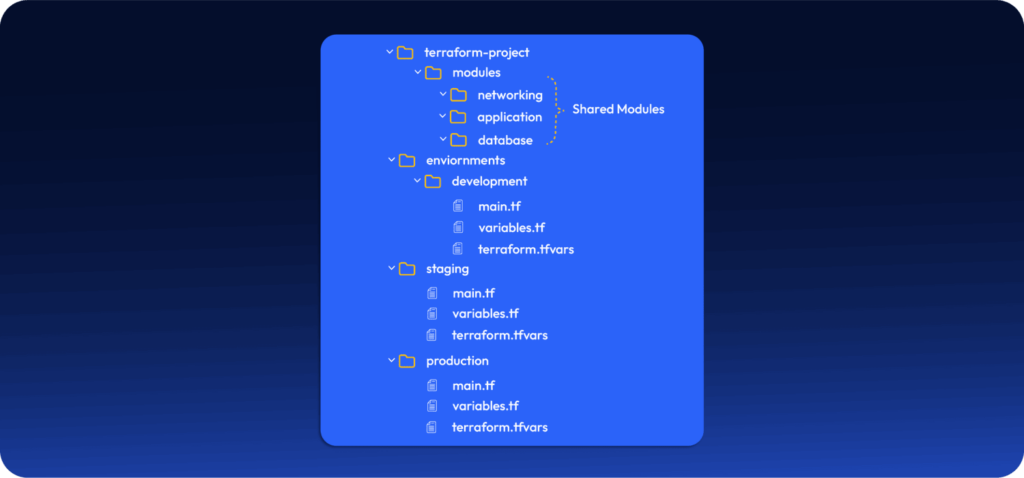
With this structure, each environment has a separate backend configuration:
- environments/dev/main.tf
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
terraform { backend "s3" { bucket = "company-terraform-states" key = "dev/terraform.tfstate" region = "us-east-1" encrypt = true use_lockfile = true #S3 native locking } } module "database" { source = "../../modules/database" environment = "dev" instance_size = "t3.small" # Other dev-specific configurations } |
- environments/production/main.tf
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
terraform { backend "s3" { bucket = "company-terraform-states" key = "production/terraform.tfstate" region = "us-east-1" encrypt = true use_lockfile = true #S3 native locking } } module "database" { source = "../../modules/database" environment = "production" instance_size = "m5.large" # Other production-specific configurations } |
Note: The above example only uses hardcoded values for illustration. Use variables from variables.tf instead of hardcoding values. This directory structure increases code repetition since you repeat the main.tf and variables.tf files in each environment. However, you can minimize repetitions by using shared modules. This tradeoff is worth it for environment isolation.
5.2 – Terraform Workspaces- Less recommended
Terraform workspaces lets you manage multiple environments using the same Terraform configuration files. When you create a workspace for each environment (like dev, staging, production), Terraform automatically manages distinct state files for them, whether you store them locally or in a remote backend like S3 if you use terraform with AWS.
One of the more common Terraform errors we see is teams misusing or over-relying on workspaces—especially when managing multiple environments.
Here’s how Terraform workspaces are typically used — and why they might not be the right fit for every use case:
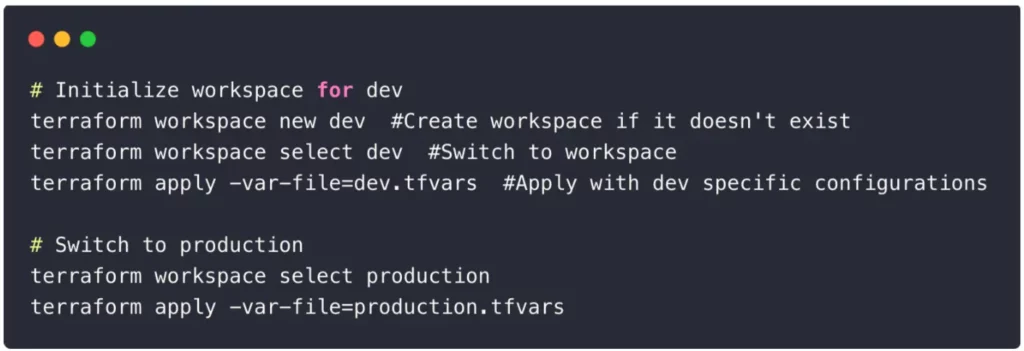
Additionally, the current workspace name is available as a variable in your Terraform configuration. You can use it to make conditional changes to your infrastructure.
1 2 3 4 5 6 7 8 9 10 |
resource "aws_instance" "controlmonkey" { count = terraform.workspace == "production" ? 5 : 1 instance_type = terraform.workspace == "production" ? "m5.large" : "t3.micro" tags = { Environment = terraform.workspace Name = "${terraform.workspace}-instance" } } |
Of these two methods, use the workspace method only if changes across your environments are minimal. Terraform workspaces can lead to more complicated resource configurations as you rely heavily on conditional logic. Also, they store all environments’ state files in a single backend (Everyone who needs to manage dev environments also has access to the production state).
At the same time, managing environment isolation manually can quickly become complex in a multi-cloud setup. Platforms like ControlMonkey help to implement these patterns at scale.
6. Skipping Automated Testing
Without testing, misconfigurations with security implications (S3 bucket with public access), standard violations (S3 without versioning, Security Groups that open all ports) & logic flaws can creep into deployments.
Tools like Terratest help you address these challenges in both AWS and Azure infrastructure by programmatically validating your Terraform configurations before they reach production.
Terratest can raise different status codes so your automated pipelines can fail when tests fail.
Here is a sample terratest script which validates a bucket name & checks if the bucket has versioning enabled;
Improving on security
Beyond functional tests, it’s essential to automate security checks. We’ve seen many teams start without security checks, and when the time comes to make their infrastructure compliant with different benchmarks or standards such as CIS, PCI-DSS, HIPAA, GDPR, and more—they have to rewrite entire modules.
Checkov is an open-source static analysis tool for Infrastructure as Code that identifies misconfigurations and security vulnerabilities. You can quickly install Checkov using the command pip install checkov and run checks against your code with the command check –directory /path/to/terraform. You can integrate it into your existing pipelines to prevent vulnerable configurations in your infrastructure.
7. Terraform Errors Caused by Inconsistent File Structures
One of the most common Terraform Errors teams make is cramming numerous resources, data sources, and variables into a single monolithic .tf file. This approach might seem convenient initially, but as your infrastructure expands, it becomes increasingly difficult to navigate, troubleshoot, and collaborate effectively.
A well-structured Terraform project typically includes several specialized files, each with a distinct purpose.
├── main.tf # Provider configuration (AWS or Azure)
├── variables.tf # Input variable declarations
├── outputs.tf # Output value declarations
├── terraform.tfvars # Variable assignments (gitignored for sensitive values)
├── locals.tf # Local values for code reuse
├── network.tf # VPC/Subnet or VNets/NICs
├── compute.tf # EC2 instances, autoscaling groups
├── storage.tf # S3 buckets, EBS volumes
├── database.tf # AWS RDS instances,DynamoDB tables,Azure Cosmos DB etc.
├── iam.tf # IAM roles, policies, users
└── versions.tf # Terraform provider constraints (AWS, Azure, or other)
8. Terraform State File Mismanagement
By default, Terraform stores the state locally in a terraform.tfstate file. You can accidentally delete, overwrite, or commit local state files to version control, exposing sensitive data. Also, your team members can’t access your state file, making collaboration impossible.
Using a remote backend brings;
- Concurrency control: State locking to prevent simultaneous operations
- Version history: Track state file changes over time
- Security: Encrypt state files in transit and at rest. You can use separate state files (or backends) for different environments.
- Collaboration: Team members can safely work on the same infrastructure
AWS S3 is one of the most popular remote backend configurations. Here’s how to set it up if you’re already using Terraform AWS provider:
1 2 3 4 5 6 7 8 9 |
terraform { backend "s3" { bucket = "controlmonkey-state-bucket" key = "path/to/your/statefile.tfstate" region = "us-east-1" encrypt = true use_lockfile = true #S3 native locking } } |
Terraform supports many other backends through different providers. If you are using Azure and store the state in Azure Blog Storage, similarly you can implement state locking using Azure Compos DB.
Therefore, if you are deploying through Terraform to multiple clouds, a cloud-agnostic service could be your best bet.
9. Committing Sensitive Files
Pushing credentials, API keys or state files containing infrastructure secrets to Git repositories permanently adds them to the repository’s history, making them difficult to remove—even after deletion. The simplest yet most effective mitigation is implementing a proper .gitignore
To prevent accidental commits of sensitive or environment-specific files, use the following .gitignore setup for your Terraform projects:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
# Local .terraform directories **/.terraform/* # .tfstate files *.tfstate *.tfstate.* # Crash log files crash.log crash.*.log # Exclude local execution environment configuration which can lead to inconsistencies .terraform.lock.hcl # Ignore CLI configuration files .terraformrc terraform.rc # Ignore local environment variable files .env .env.* # Ignore SSH keys *.key # Ignore provider credentials and config files .aws/ .gcloud/ .azure/ # Ignore plan files *.tfplan |
10. Final Terraform Error: Overcomplicating Configurations
Effective Coding Patterns
Use Consistent Variable and Resource Naming
Avoid 👎: Inconsistent, unclear naming.
Inconsistent, unclear naming
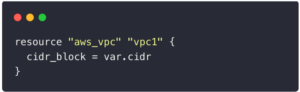
Good 👍: Descriptive naming with a consistent pattern
Descriptive naming with consistent pattern
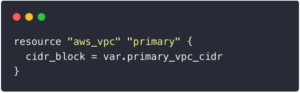
- Use meaningful names for variables.
- Add descriptions when you define variables in variables.tf so documentation tools can automatically generate good documentation.
- Use proper variable types.
- Use locals when complex conditionals are required.
Don’t deep nest modules
Avoid 👎: Nested Modules
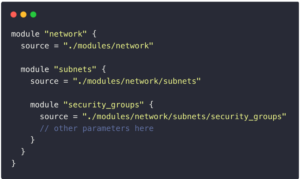
Good 👍: Flat module structure
Don’t use excessive conditional logic
Avoid 👎: Embedding too much conditional logic within Terraform configurations.
Good 👍: Using locals to calculate values first
Effective Formatting & Documentation
Terraform files can be automatically formatted using the terraform fmt command. This leads to more readable code and improves formatting consistency.
Each module should include a well-structured README.md. Terraform documentation typically follows a template which includes title, overview, usage, inputs, outputs & additional details. A sample markdown is as follows;
We recommend automating the maintenance of your Terraform documents using a document-generating tool such as terraform-docs.
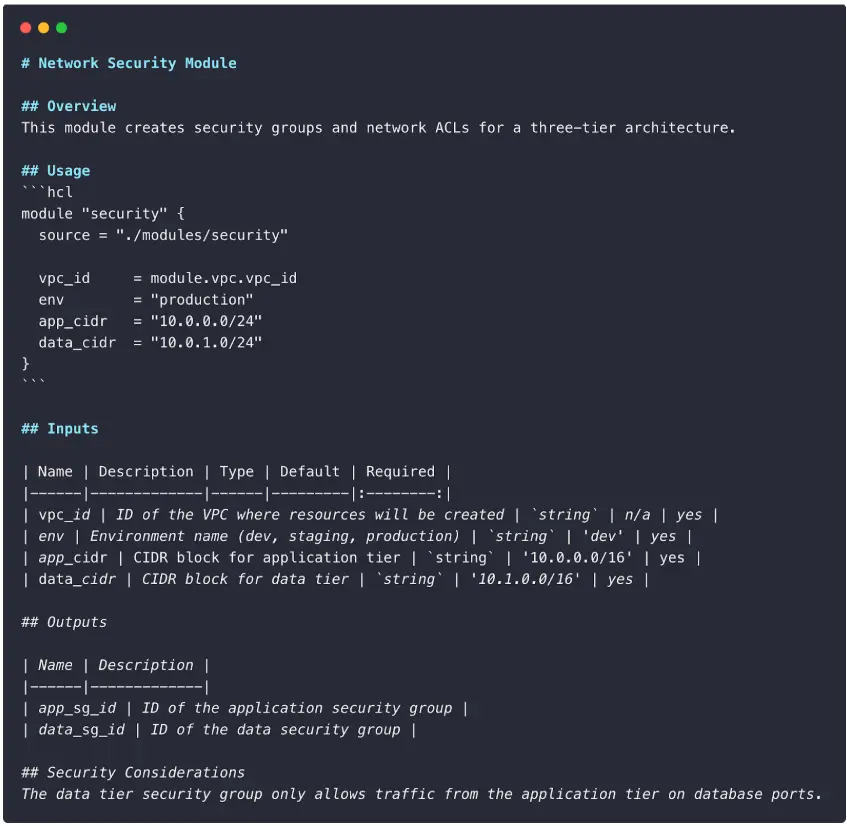
Final Thoughts on Terraform Errors
Terraform is a powerful tool for infrastructure management. However, mastering it requires attention to detail.
If you are looking for a way to enforce these practices in multi-cloud environments, platforms like ControlMonkey, can help to implement it with minimal complexity.
By avoiding these common mistakes, teams can build robust and maintainable infrastructure. Remember that effective Terraform implementation isn’t just about technical correctness; it’s about creating a sustainable process that supports collaboration, ensures security, and accommodates growth. The time invested in setting up proper workflows and processes today will result in a huge return in the future.
Ready to improve your infrastructure management? Book a demo with ControlMonkey today to see how our platform can streamline your Terraform workflows.

How to Troubleshoot Terraform Deployments on AWS and Azure
To debug Terraform on AWS, start by checking the Terraform state file with terraform state list, and use TF_LOG=DEBUG to view detailed logs. Review AWS IAM permissions and resource quotas as well — these are common root causes of Terraform deployment failures.
Use terraform plan to preview the dependency graph, and add depends_on explicitly when needed. Break large configurations into smaller modules to reduce hidden dependencies and improve maintainability.
This often happens when the state file is out of sync. Use terraform refresh or manually import missing resources using terraform import. Avoid making changes via ClickOps, which can cause these mismatches.
ControlMonkey automates drift detection, compliance scanning, and Terraform error resolution. It monitors state file inconsistencies and provides real-time visibility into infrastructure issues before they impact deployments.
A cycle error in Terraform means there’s a circular dependency between resources. This usually happens when two or more resources refer to each other directly or indirectly. Use depends_on to fix the creation order, or refactor your configuration into modules to eliminate the cycle.
This usually happens when $ is used incorrectly outside of interpolation syntax. Terraform expects variables to be referenced like ${var.name}. Using raw $ symbols in strings without escaping them can trigger an invalid character error.